Introduction:
As developers, we often face the challenge of ensuring that our applications are secure. One of the ways to achieve this is by using JSON Web Tokens (JWT) to authenticate and authorize user requests. JWTs are a popular method for token-based authentication, as they are easy to use and secure. However, in some cases, we may need to add an extra layer of security to our JWTs. In this blog, we will discuss how to encrypt and decrypt the payload of a JWT token for extra security in Node.js.
What is JWT?
JSON Web Tokens (JWT) are a compact, URL-safe means of representing claims to be transferred between two parties. JWTs are often used for authentication and authorization purposes in web applications. A JWT consists of three parts: a header, a payload, and a signature.
JSON Web Tokens (JWT)
What is Encryption and Decryption?
Encryption is the process of converting data into a secret code to protect the data’s confidentiality. Decryption is the process of converting encrypted data back to its original form.
Why Encrypt and Decrypt JWT Token Payload?
Encrypting the JWT token payload provides an extra layer of security to the token. It ensures that the data within the token remains confidential and cannot be read by anyone except the intended recipient. Decryption, on the other hand, allows the recipient to read and understand the contents of the token.
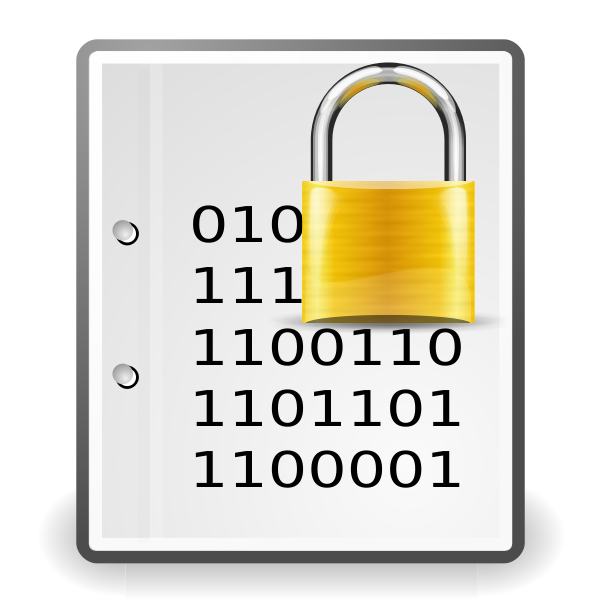
Here’s an example of how to encrypt the payload of a JWT token:
const jwt = require('jsonwebtoken');
const crypto = require('crypto');
// create a secure encryption key
const secret = crypto.randomBytes(32);
// create the payload
const payload = {
user: 'Alice',
email: 'alice@example.com'
};
// encrypt the payload
const encryptedPayload = crypto.createCipheriv('aes-256-cbc', secret, '1234567890123456').update(JSON.stringify(payload), 'utf-8', 'base64');
// sign the token
const token = jwt.sign({ payload: encryptedPayload }, 'your-secret-key');